Building a simple Form app
Using Pages, Functions and Javascript to build a customized app.
Starting out with a simple Form
When creating Apps inside Jestor, you're usually going to break them down into three main components:
- The standard page: what the user will see as soon as he opens the app.
- Javascript functions: what will happen as the user interacts with the app.
- Standard functions: back-end functions that do things like search for information or create records. They may also return front-end values.
So, in the case of a simple form to create a record in a table, you're probably looking at:
- A page with input fields and a Submit button.
- A JS function to get the information from the fields and call a standard function.
- A function that creates a new record in a table through a Jestor.create().
First we're going to build a very basic form: a text input and a submit button. This will create a company in a Companies table and display a message confirming the record was created, as well as displaying the ID of this new record.
Building the app
First things first, we'll create a page called CompanyForm. We'll create the HTML for the form, which we can write in the Front-End part of the Page. In it, we'll have an input field and a button.
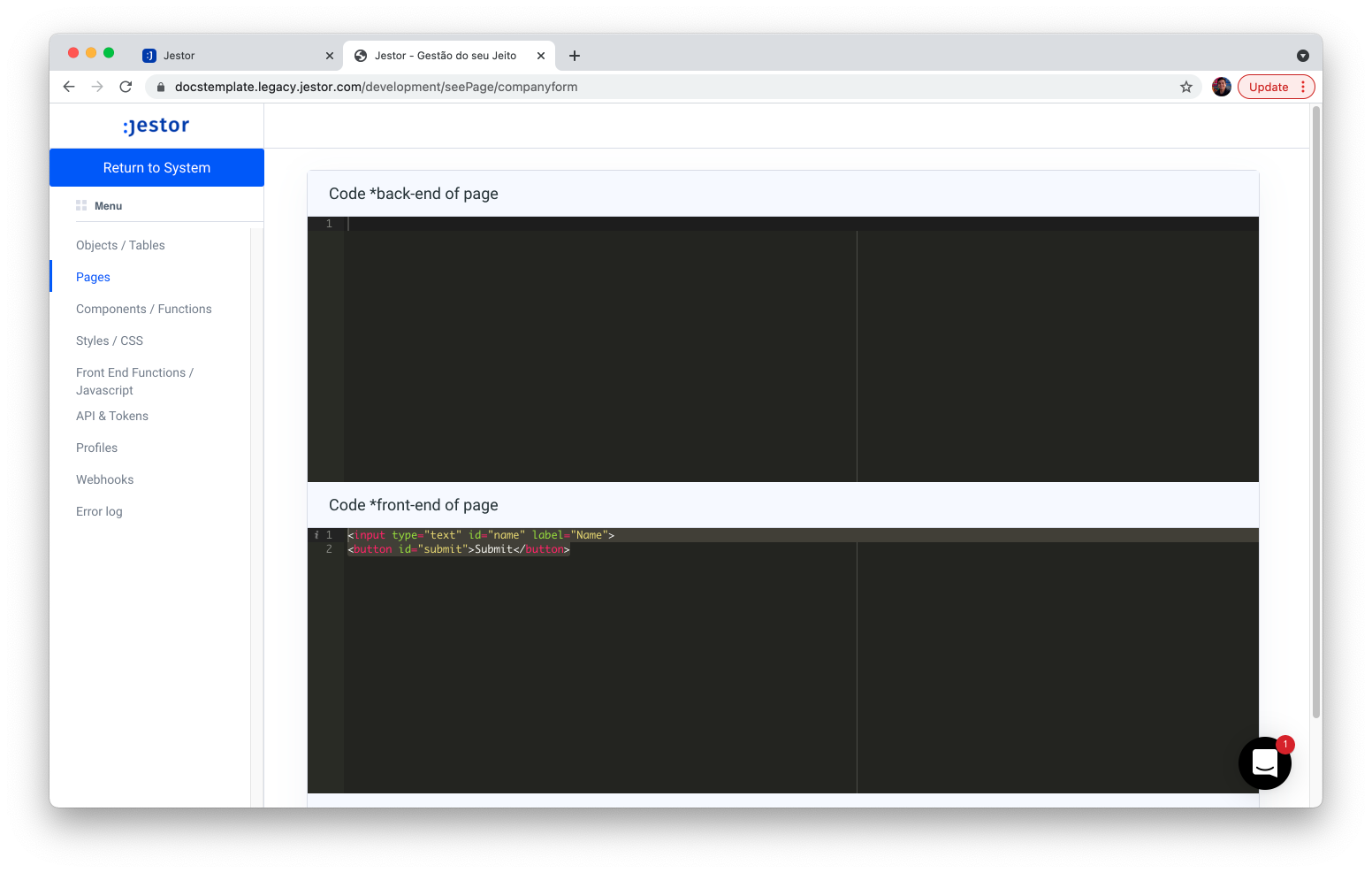
<input type="text" id="name" label="Name">
<button id="submit">Submit</button>
The result is this page:
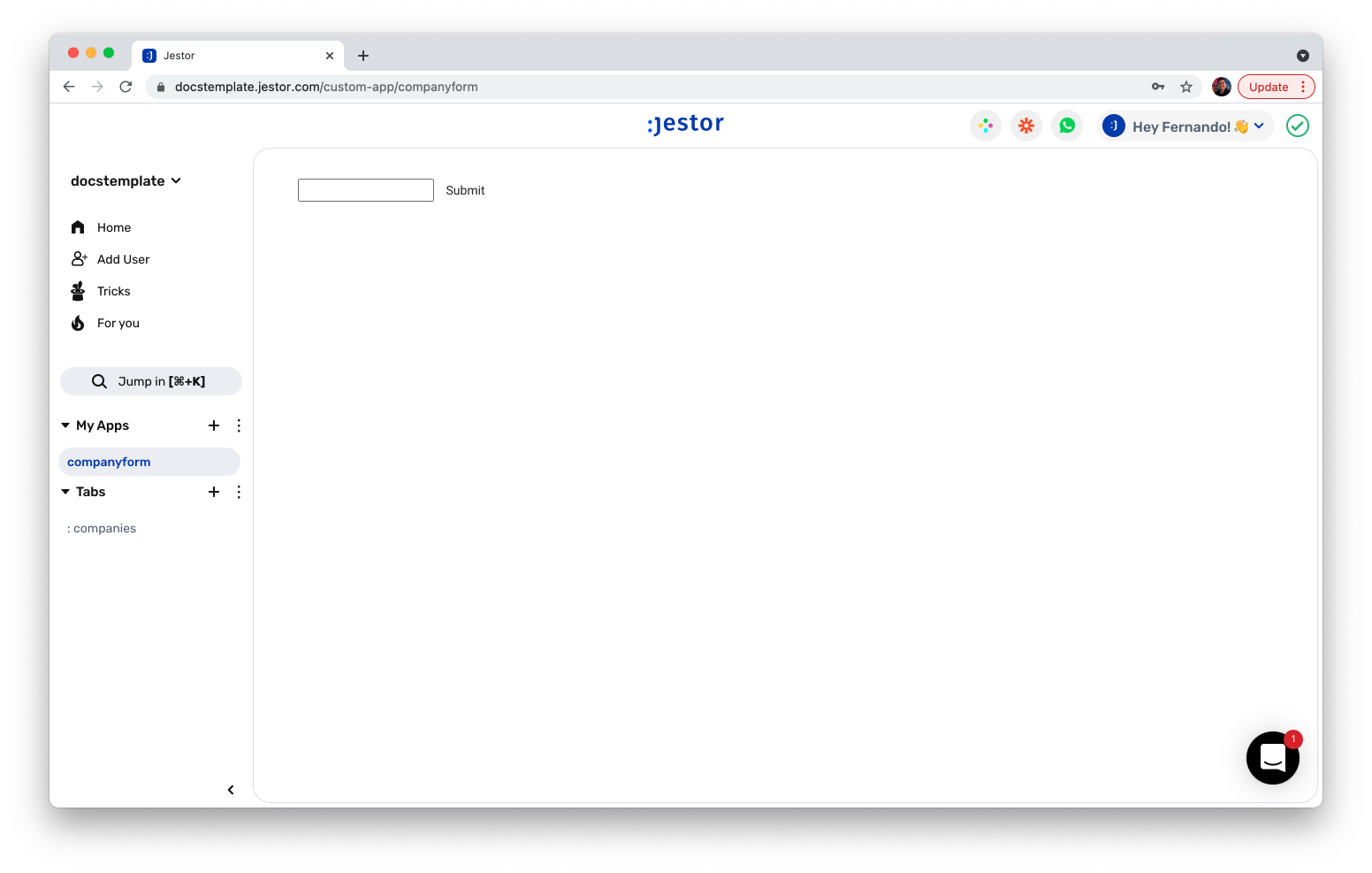
Now we're going to create a JS function called SubmitForm that is activated when the Submit button is clicked. It's going to call a standard function called CreateCompany (which we haven't created yet, but we'll get around to that).
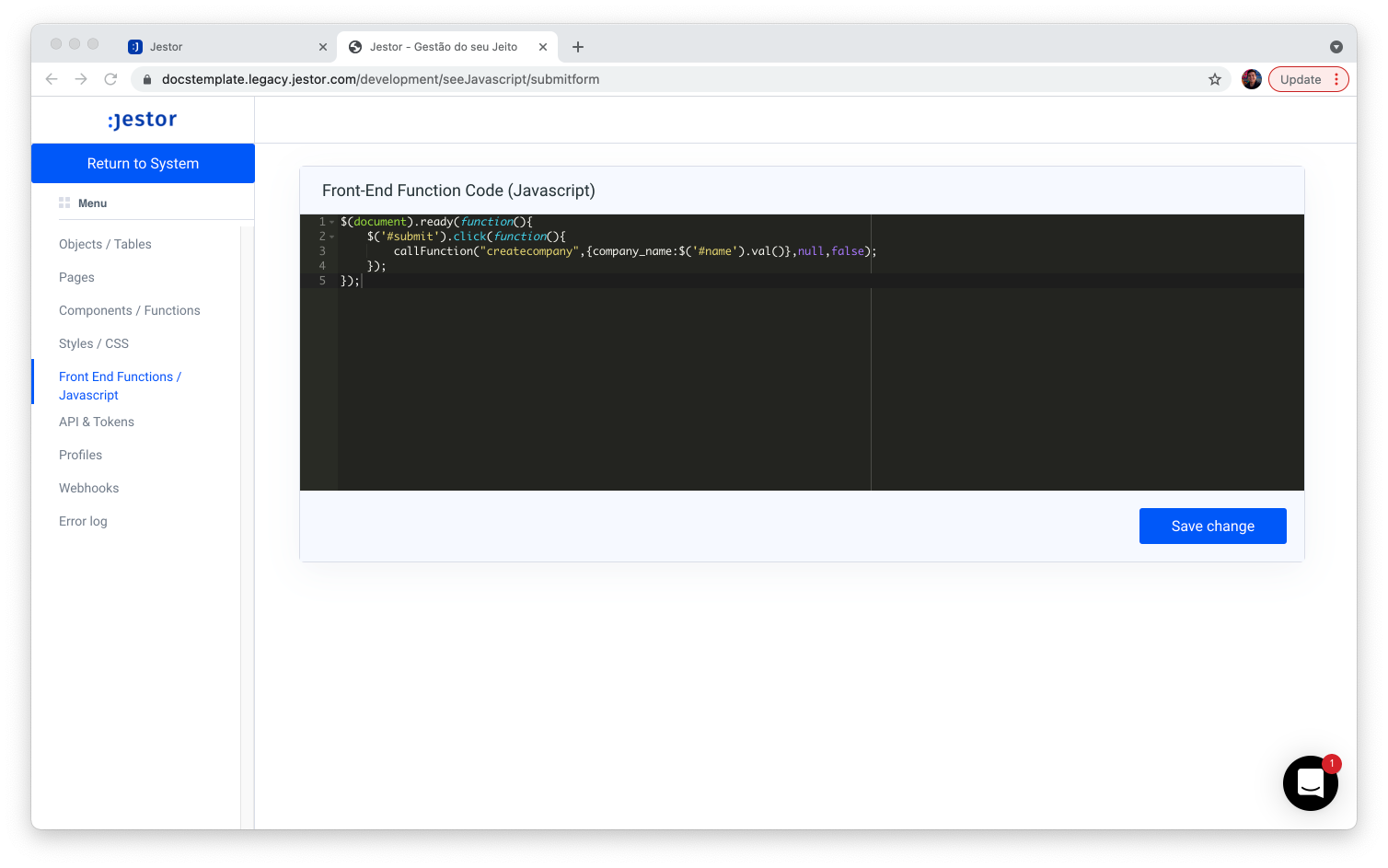
$(document).ready(function(){
$('#submit').click(function(){
callFunction("createcompany",{company_name:$('#name').val()},null,false);
});
});
As you can see, when we've built this function in a way that, when the submit button is clicked, it's going to call a createcompany standard function, sending the text on the name field of the page as the variable company_name.
Now, we're going to create the standard function createcompany. This function will use the information on $data['company_name'] (that is, what it's receiving from the form) to create a company record.
We'll also go ahead and create a message to be displayed as feedback, and return it through the Front-End part of the function by using {{}} to access the Back-End variables.
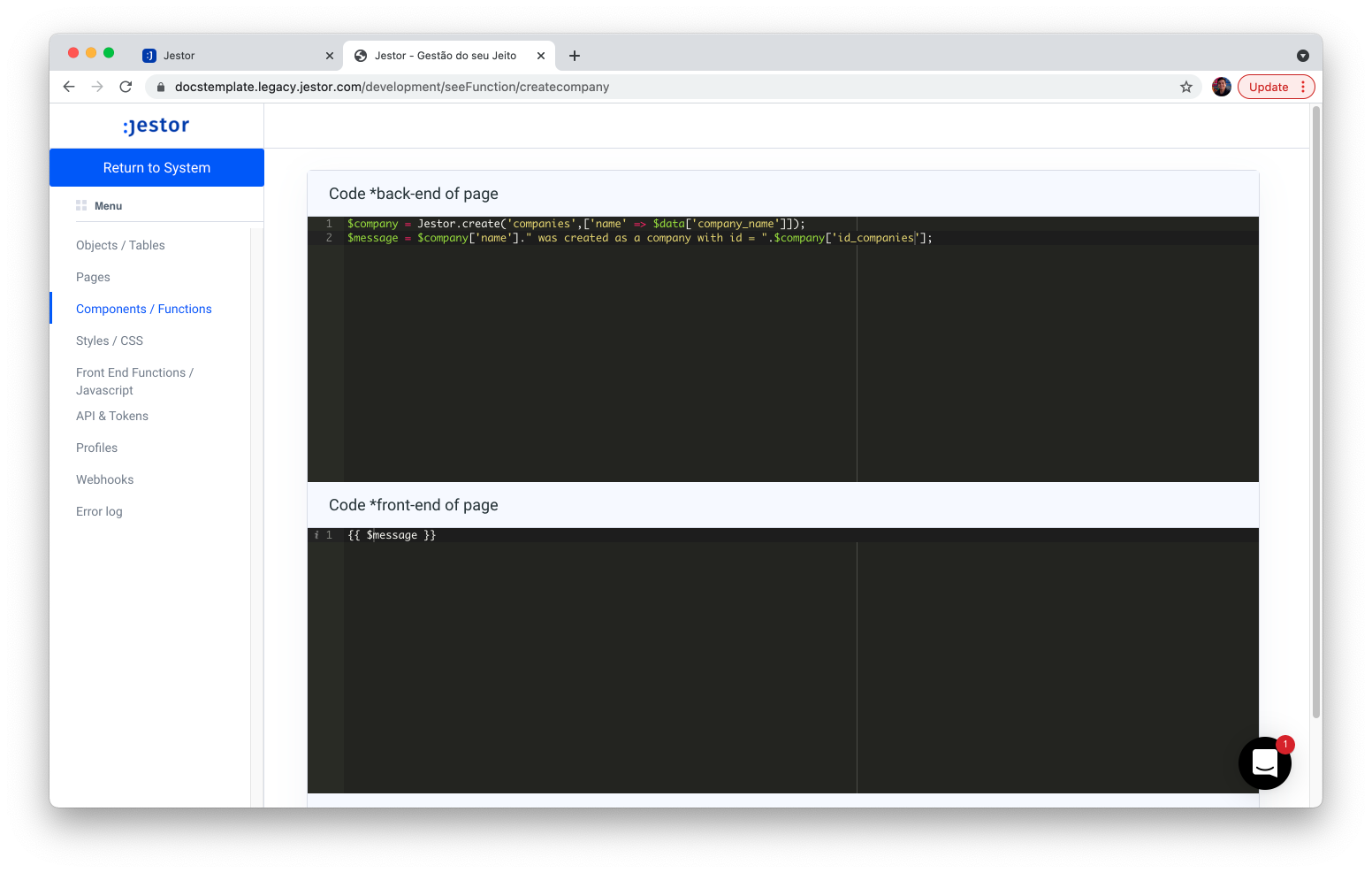
$company = Jestor.create('companies',['name' => $data['company_name']]);
$message = $company['name']." was created as a company with id = ".$company['id_companies'];
{{ $message }}
Now, we're going to go back to the page itself and add an element where we can render this message. We're also going to add the Javascript function to the page SubmitForm.
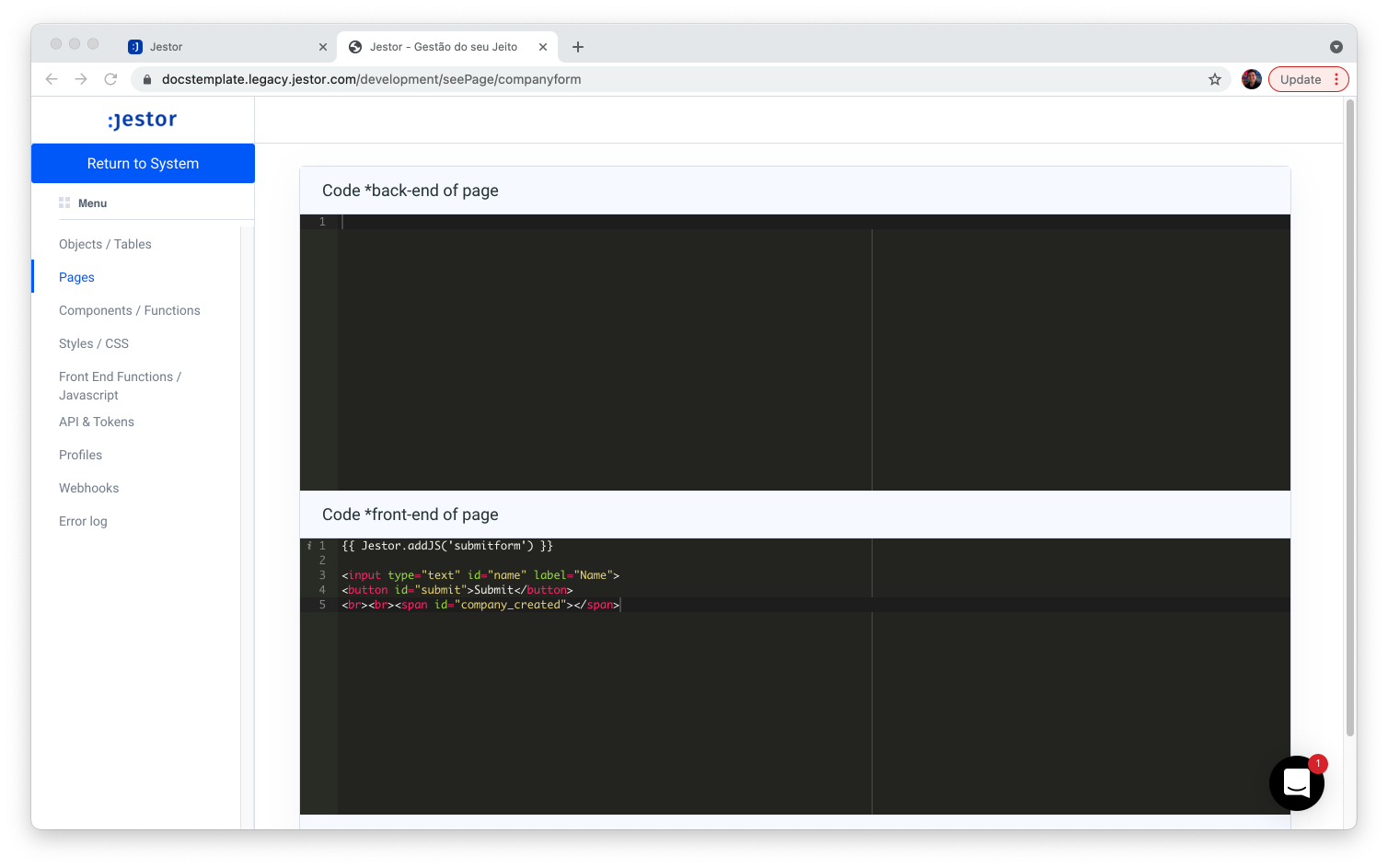
{{ Jestor.addJS('submitform') }}
<input type="text" id="name" label="Name">
<button id="submit">Submit</button>
<br><br><span id="company_created"></span>
Finally, we're going to modify the JS function SubmitForm so the result we get from the standard function CreateCompany (that is, $message), is rendered on the new HTML element. We can do that by passing the HTML element ID and setting reload to true.
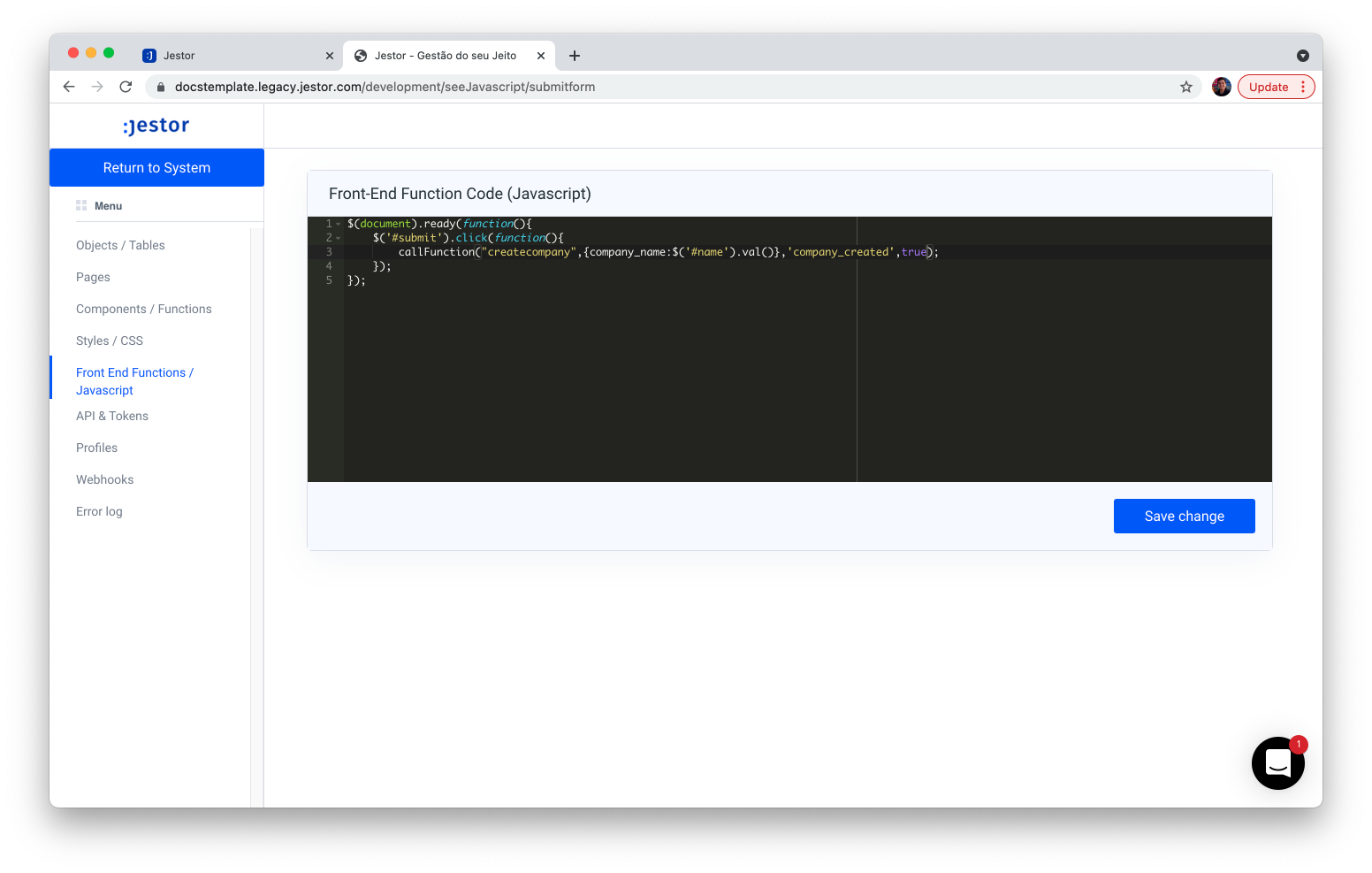
$(document).ready(function(){
$('#submit').click(function(){
callFunction("createcompany",{company_name:$('#name').val()},'company_created',true);
});
});
Alright! So now let's test this new app:
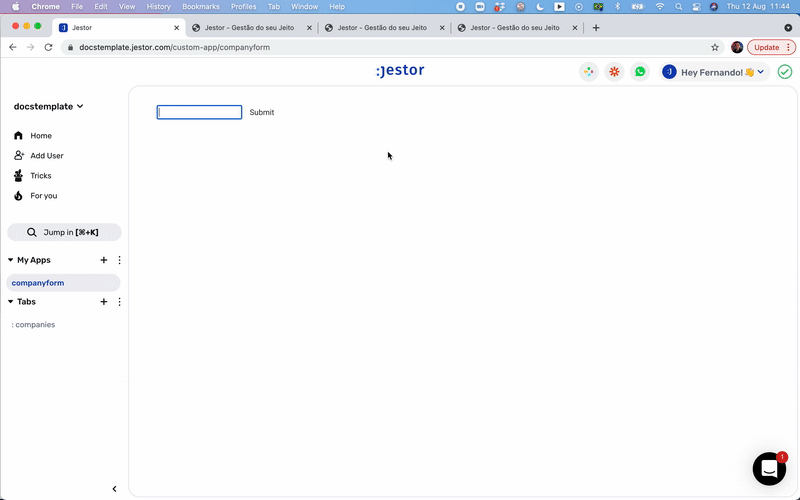
Success 🎉
Everything is working as intended!
Create forms with individual elements
Be aware that using an HTML form tag on Jestor won't work appropriately: create each field as an individual element, as we did in this example.
Updated almost 4 years ago