My first automation
A simple tutorial to get things going!
A simple structure
For this example automation, we're going to automatically create Clients records when a Lead on a Kanban reaches the "Won" status.
Start by creating a table called Leads with three fields:
- Name: this field is already created by default.
- Deal Value: currency type field.
- Status: single selection type field, with New/In Progress/Won options.
- Now click on "Kanban", select the "Status" field and press "Create" to turn this table into a Kanban.
- Then, create a Clients table with the following fields:
- Name: this field is already created by default.
- Lead: connect tables field pointing to the Leads table.
- Status: single selection type field, with Active/Inactive options.
Creating an automation
Now we're going to create our automation.
- Go to the Developer Area, find the Leads object, click on it and go to the bottom of the screen
- Click on the Leads table
- Go to the bottom of the screen
- Click on "+ Create trigger" to name your trigger and start coding.
Now, let's think about the Trigger itself. We want to create a client when a lead reaches "Won" status. We can assume the lead existed before in other statuses, so what the user will be doing is probably dragging a card to the "Won" stack, which means he'll be updating the record.
In this case, it doesn't matter if the automation runs before or after the record is update, but choose only one of those triggers so the automation doesn't run twice.
As for the automation itself, we want the following to occur:
- The automation should run when the lead reaches "Won" status.
- However, the automation should only run when the status changes to "Won". If the card is already in "Won", updating it should not create the client again.
- Should the conditions above be met, create a client that's connected to the lead.
Our code will look something like this:
if($objectNew["status"] != $objectOld["status"]){ #if the new status is different from the old status, that is: the status has changed
if($objectNew["status"] == "Won"){ #if the new status is "Won"
$newclient = array(
"name" => $objectNew["name"], #the new client will have the lead's name
"lead" => $objectNew["id_leads"], #the new client's lead field will connect to the lead's record (connect tables type fields always use id)
"status" => "Active" #the new client will have "Active" status
);
Jestor.create("clients",$newclient); #creates a client with the information conatined on $newclient
};
};
Just press the "Save changes" on the bottom of the screen to save your automation.
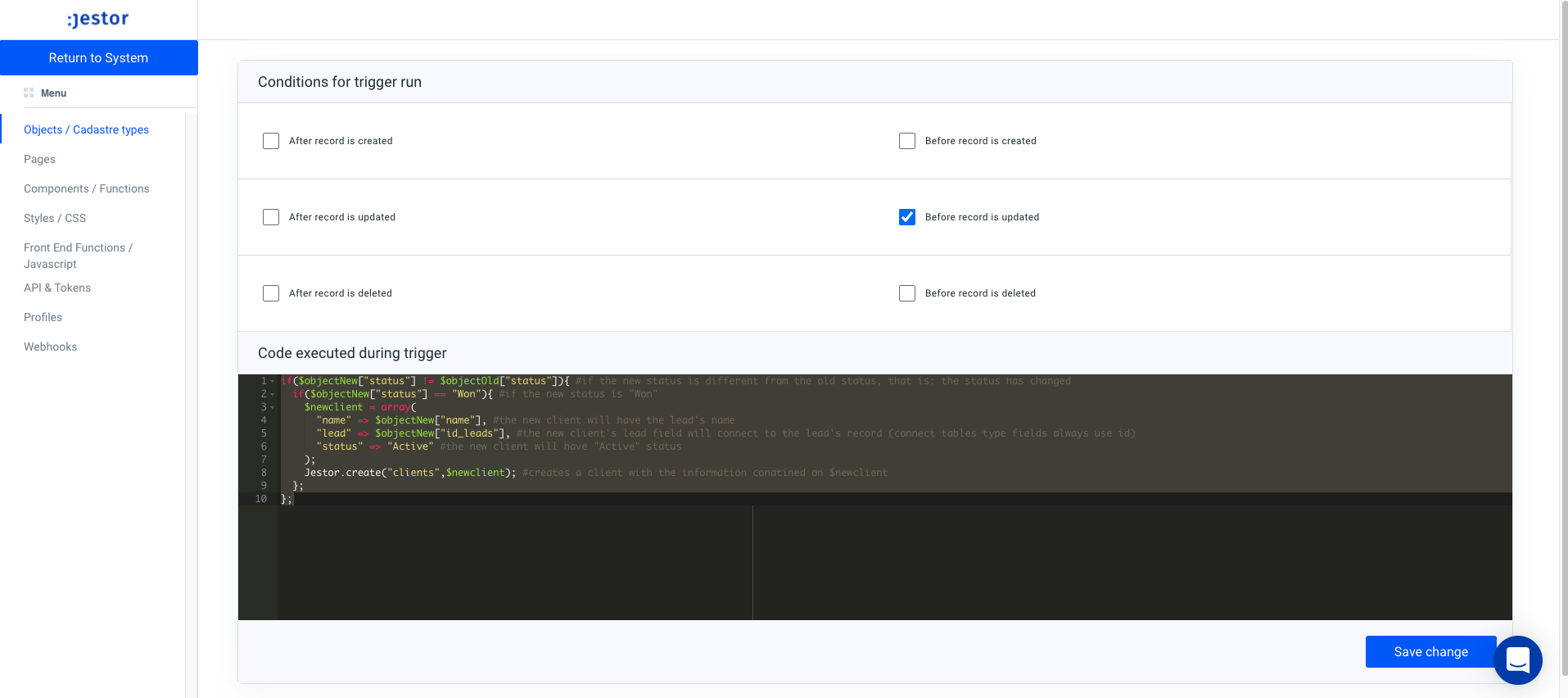
Saving the automation
And now, when a lead is dragged to "Won", a connected client is automatically created!
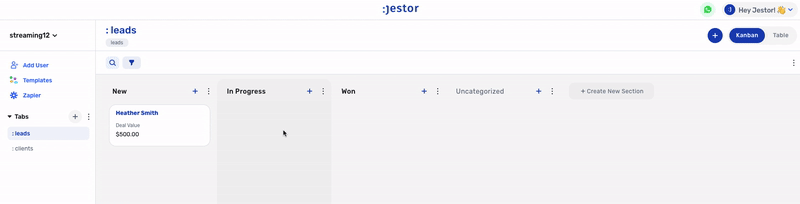
Automation complete 🎉
Of course, automations can be much more complex than this. Using your imagination and a little bit of code, you can create anything you want :)
Loops and Conditions
The more you know about programming, the more you can build on jestor's developer area. However, we find that just knowing about operators, If conditions, and For, For Each and While loops are enough to empower most users to do amazing automations. You can learn more about them below:
Operators: https://www.php.net/manual/en/language.operators.php
If: https://www.php.net/manual/en/control-structures.if.php
For: https://www.php.net/manual/en/control-structures.for.php
For Each: https://www.php.net/manual/en/control-structures.foreach.php
While: https://www.php.net/manual/en/control-structures.while.php
Updated about 1 year ago